There’s a lot of content around Java interview questions for 2022 or for the previous years. I genuinely think most of that content is written by people never ever doing interviewing.
I had 2 articles already what Java interview questions you should not ask in 2022 if you’re an interviewer:
- The Java interview questions you should not ask in 2022
- The Java interview questions you should not ask in 2022 Vol. 2
In this article, I’ll show you a couple of my Java interview questions for 2022 along with my opinions.
Let’s begin.
Evaluation criteria
Before I start talking about some specific interview questions we gotta discuss what’s the purpose of an interview and what kind of things you should look for.
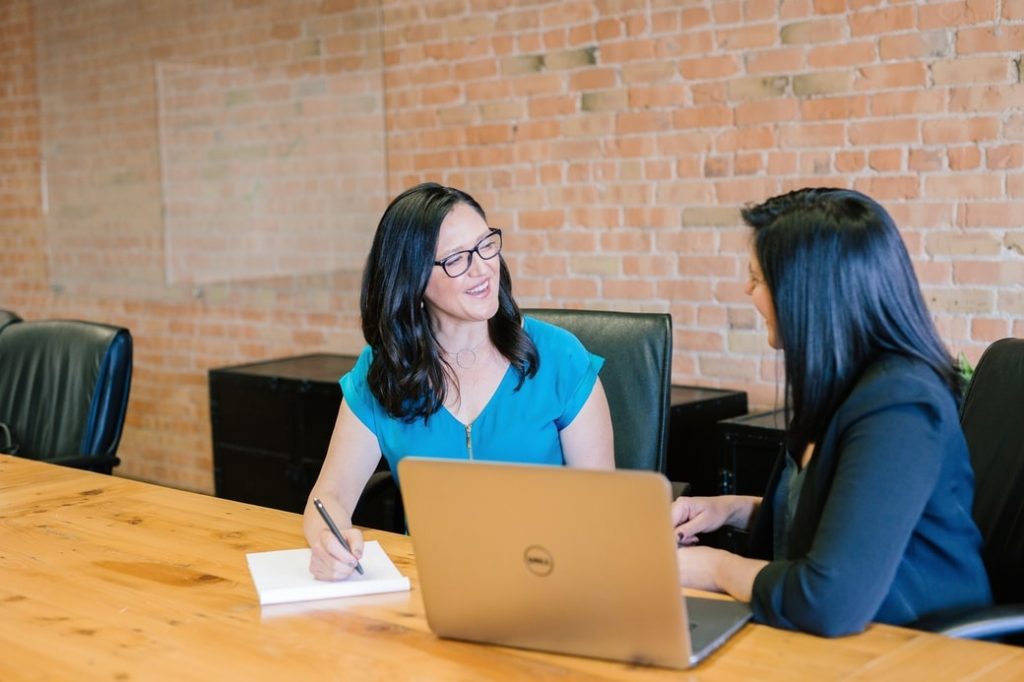
Nowadays it’s almost impossible to work alone on a project. You usually have a team of people you meet daily, talk to, ask for help, etc. This team may consist of 3-10 people, depending on the specifics of the project.
One of the first evaluation criteria for any people is the ability to communicate. I’ve seen superheroes delivering good solutions on time to difficult problems, yet the team got frustrated because nobody knew what he was doing, what approach he’s choosing and how the solution is going to be maintained on the long run.
Communication is #1. I don’t think we have to be experts at putting words nicely – especially if you need to speak on a non-native language – but making sure others understand you is key. Communication doesn’t only mean how well you know/can use the language.
Agile teams have daily standups to talk about 3 things.
- What did you do between now and the last standup?
- What will you do between now and the next standup?
- Is there any impediment you need help with?
If people can’t/don’t want to explain they hit a roadblock they need help with, or they can’t explain what they were doing yesterday. That’s a huge issue. We gotta talk to and help each other along the way. We’re in the same boat.
So that’s my topmost element in the list. Next up, and this is actually the last for me. The ability to think. I’m not interested at all whether somebody can tell me how a HashMap is implemented in Java; or why generics are contravariant in Java. These are good if somebody knows them but it’s lexical knowledge for most of the projects and that’s totally learnable. I mean I can imagine a project where you have to reimplement the HashMap to provide a tiny bit better performance but come on, that’s usually not what projects need.
I rather like to test interview candidates with open ended questions and see their thought process. Sometimes I also drop totally unexpected questions to see the reaction of a candidate and how they can adjust themselves to the newly created environment; because trust me, when there’s a production incident call with 5-6 stakeholders and the candidate freezes, that’s usually not going to end well.
Couple of specific questions
As for everything, there’s no silver bullet for interviewing either. There’s no predefined list of questions you should ask in every scenario. Don’t be afraid to experiment and try new things. I’ll give you some of my questions that I usually tune to a certain environment.
Let’s begin with the questions.
Migration from Cassandra to PostgreSQL
The question is this: There’s a project running for a couple of months, 30 people working on it and the app is using Cassandra as a data store. The product is not yet in production. The client comes in and says we need to go into production in 2 months. Your reaction is, well, that’s doable, we’re prepared for it. We’ll need to do a couple things but it’ll definitely fit into the 2 months schedule. Then the client says, yeah but we got a good deal at a cloud provider that doesn’t have Cassandra but it supports PostgreSQL. You’re the engineer to decide what to do next. What would you do?
That’s it. That’s the question. See how open ended it is? I’ve asked this question over a 100 times at least and I’ve never ever got the same answer to it.
You can focus on a lot of things like:
- How the hell are you gonna rewrite the whole data access layer to PostgreSQL on time
- If the rewrite is somehow done, how the hell are you going to make sure everything is working
- If you’d ask the client to look for other alternative cloud solutions with C* support
- The differences between C* and PostgreSQL; and why the rewrite could be difficult
And these are just starting points for a broader discussion. Whatever the candidate brings up, I dig into it. If they start talking about the technological aspect of for example using Spring Data for the Cassandra layer initially, it’d be like minimal change to the code to switch to Spring Data JPA. We go deeper and deeper, ask more questions.
If they start taling about testing, let’s dig into testing. What testing process they imagine, where they’d draw the line between enough and not enough test coverage and stuff like that.
If they’re good talkers, they might start to discuss strategies on how to make the client change which cloud provider to use. Showing a cost evaluation on doing the migration versus just going with another cloud provider.
Whatever it is, I dig deeper and ask other open ended questions to lead a whole discussion to see their thought process.
I often vary this question and instead of Cassandra/PostgreSQL specifically, I ask them what NoSQL/SQL DB they have XP with and compare those 2. Like MongoDB to MySQL.
How would you start a brand new project?
This is a multi-layered question. You can totally focus on your organization’s practice but I like to see how a candidate would start a completely new project from scratch if they’re the ones choosing the technology.
For example a common answer here is, Java + Spring Boot + Hibernate combo. And then I usually ask why would they choose that. This just made it a whole other question we started from. We can discuss why the candidate thinks Spring Boot is a good project starter. They can talk about the framework itself, they can talk about the mass adoption of the framework in the industry, they can talk about the fact that hiring is probably more easier this case in the future than choosing an exotic framework, whatever. This can bring in more and more questions, you just gotta find them in the candidate answers’.
Hibernate is the same. I’m a big believer of JPA and Hibernate personally but I know it has its place. And I simply not choose it for most projects because it’s fairly easy to end up with serious performance problems if the developers are not careful. But this is another aspect you can ask, if the candidate had any performance issues when using Hibernate.
How would you debug a performance issue?
The question is this: You have a web UI, a backend and a database for data storage. The Product Owner creates a new bug ticket in JIRA saying that if he clicks on a button on the page, it takes 5 seconds until he gets the notification message that the operation was successful. How would you debug this?
This is again very much an open-ended question with multiple focus points:
- Somebody starts with talking about the quality of the JIRA ticket
- Somebody starts analyzing the problem from the frontend to the backend
- Somebody would talk about lack of testing and the fact that this was caught by a user instead of an automated test
- Somebody starts discussing the monitoring and tracing tools available
If the candidate talks about the JIRA ticket, let’s discuss how a good bug report would look like and what kind of info should be there. If that’s set, how would you enforce bug reports to have certain quality requirements.
If they start analyzing the problem, we often talk about the process they break it down to actionable pieces. Like how to check the frontend, whether it’s slow there. Then how to check the backend and what parts are slow. How to analyze/check the DB queries if they are slow, etc.
If they talk about testing, let’s discuss testing then. It’s also a very interesting point because performance testing is not black and white like a unit test and there are a lot of questions to talk about.
How to handle personal conflicts during a code review?
The question is built on the idea of having disagreement during a code review between 2 developers. Let’s say one dev submits a PR that the other needs to check. The other dev puts some comment there and the author doesn’t really agree with the comment and takes it personal. How would you handle it? I usually focus on 2 different roles with this question, either you’re a senior dev who has to help come to an agreement between the 2 devs or you’re the dev who commented on the PR. Obviously the role depends on the job I’m interviewing the candidate for.
This is again a very open ended question and there’s no perfect solution to it. But it definitely takes a good communicator to handle these kind of situations.
Based on what attributes would you choose a data-access framework?
Would you go with JPA + Hibernate? Would you go with jOOQ? Would you go with MyBatis? Would you use Spring Data JPA? Would you write a custom data access layer?
There are a lot of questions that could arise from these options. Basically the first question I ask if somebody rapidly says “X” is “Why X?”?
There are plenty of attributes you need to consider:
- Performance
- Maintainability
- Flexibility
- Documentation
- Security
- Industry adoption aka how easy it is to hire ppl with that skill
- Tooling
- Testability
And much more. But the next thing I ask is the “What do you mean by Z?” (Z being one of the attributes).
When would you use microservices for a project?
Even though I have my personal views on when microservices should be applied to a certain problem, I’m open to hearing thoughts from others. It’s mostly a listening game for this question.
And if there’s an interesting point the candidate makes, ask follow-up questions. There’s no perfect answer here and I’m not expecting anybody to try to repeat all the fancy properties microservices are promising (on paper) but to share genuine thoughts about the problem space.
They could talk about:
- Scaling apps & teams
- Organizational difficulties – tight schedule, incompetent management, etc.
- How and why they used microservices previously
- The technology stack they’d choose
- Potential difficulties with inter-service communication
And much more.
Takeaways
That’s it for now. This is the first batch of my questions and I intentionally didn’t give any specific answer to any of them because there isn’t. These are just points to stimulate your brain for using more meaningful questions because we’re usually looking for talented people; and by talent I don’t necessarily mean superstar coders. A team player with worse coding skills could just be as valuable.
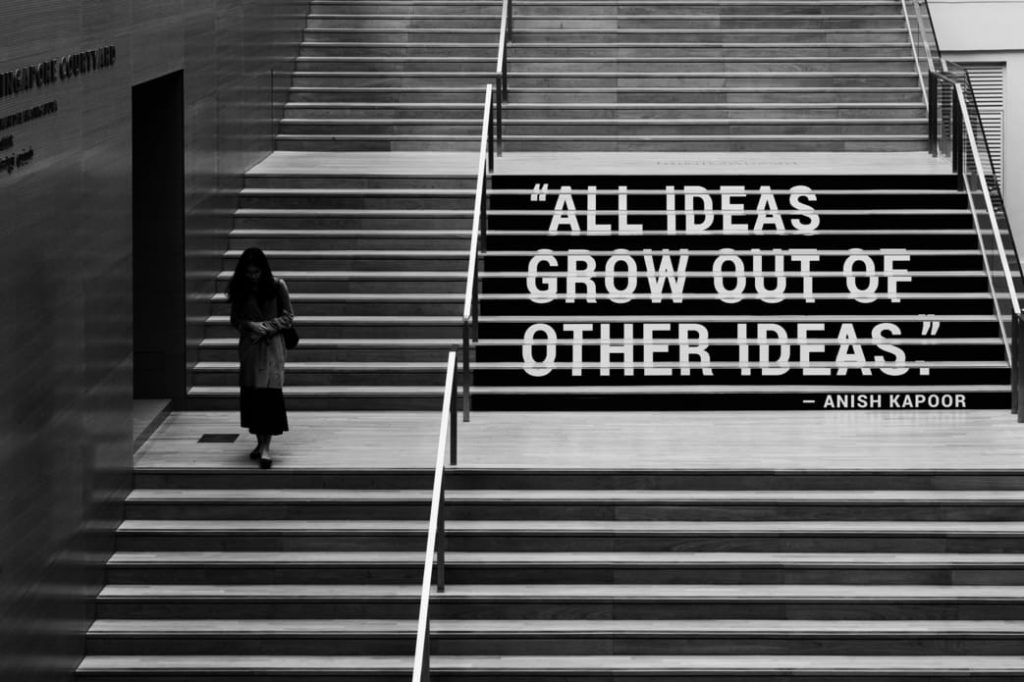
Hope you liked it, follow me on Facebook/Twitter if you want. Share it with others in the hope of stopping interviewers focusing on lexical knowledge.
Very useful points for evaluating the candidate. Many Thanks Arnold Galovics. I have taken interview of around 100+ candidates and these points definitely makes the sense.
Thanks Kailash and great to hear your feedback on this. I really think it’s critical to educate new interviewers.